Problem:
I need to apply the same validation rule to every request of my web test to test for forbidden text.
Solution:
Create a web test plug-in to insert the validation rule before each request.
Code:
using System;
using System.ComponentModel;
using Microsoft.VisualStudio.TestTools.WebTesting;
using Microsoft.VisualStudio.TestTools.WebTesting.Rules;
namespace DLBRacingTest.Custom
{
[DisplayNameAttribute("Forbidden Text")]
[Description("Adds a validation rule on every request looking for forbidden text.")]
public class ForbiddenText : WebTestPlugin
{
/// <summary>
/// Optional , delimited list of forbidden text. If left blank 'Page Error' is the only
/// forbidden text.
/// </summary>
public string Values { get; set; }
public override void PreRequest(object sender, PreRequestEventArgs e)
{
base.PreRequest(sender, e);
if (string.IsNullOrEmpty(Values))
Values = "Page Error";
foreach (string value in Values.Split(",".ToCharArray()))
{
ValidationRuleFindText pageErrorRule = new ValidationRuleFindText();
pageErrorRule.FindText = value;
pageErrorRule.IgnoreCase = true;
pageErrorRule.UseRegularExpression = false;
pageErrorRule.PassIfTextFound = false;
e.Request.ValidateResponse += new EventHandler<ValidationEventArgs>(pageErrorRule.Validate);
}
}
}
}
Explanation:
While running test on my web site I noticed a test passed although one of the pages had a Page Error on it. The reason the test passed was because there were no required extraction rules, no validation rules and the paged it failed on was the intended page. However, this test obviously should have failed. The existence of the word “Error” on any of my sites pages is a failed test. Something has gone wrong and I need to be alerted. By this point I have hundreds of web test each with many request. The thought of having to add a validation rule to every request of every test was very discouraging.
I just knew there had to be an easy way to add this validation rule to every request without doing it explicitly. A web test plug-in actually has callback that is called before every request of your test called PreRequest. So I simply add the validation rule when this callback is called. Now I only have to add a single web test plug-in and every request of that test now has a validation rule that will fail if any forbidden text is found.
I added an optional parameter to the web test plug-in called Values. If you leave it blank a single validation rule is added that searches for the text “Page Error” and fails if it is found. If you have other forbidden words you would like to search for simply set Values to a comma delimited list of values (i.e. “Page Error,Invalid”).
To use this web test plug-in you can either create a separate class library and reference it in your test project, or simply add this class to your test project. Once you do and build the code you can right click on the root of your web test and select Add Web Test Plug-in… from the context menu. Select “Forbidden Text” from the list and click OK.
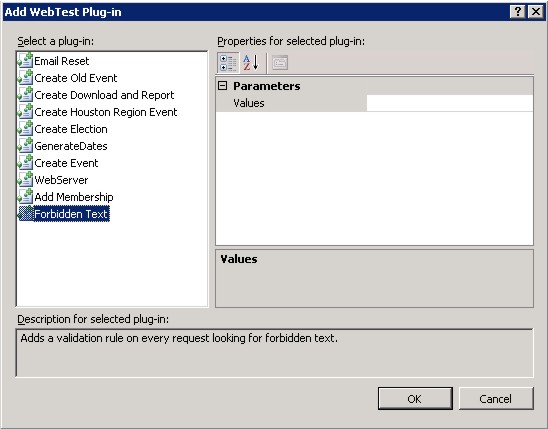